Introduction
Solutions for session 6.
Exercises
Source code for all exercises.
Exercise 1: Triangle Scan-conversion
// a,b and c are assumed to have increasing y values void scanConvertTriangle(Point a, Point b, Point c, color col) { stroke(col); // calculate slope of ab, bc and ac // run / rise because we will iterate over y coordinate double dab = (1.0*(b.x-a.x))/(b.y-a.y); double dac = (1.0*(c.x-a.x))/(c.y-a.y); double dbc = (1.0*(c.x-b.x))/(c.y-b.y); double xbegin = a.x; double xend = a.x; // from a to b for (int y = a.y; y < b.y; y++) { line((int) xbegin, y, (int) xend, y); xbegin += dab; xend += dac; } // reset xbegin explicitly to b.x // normally, this is not necessary since the first for-loop // has increased xbegin up to b.x, but in the special case // that (a.y == b.y) the first for-loop will not be executed, // so we must manually set xbegin to b.x for the second for-loop xbegin = b.x; // from b to c for (int y = b.y; y < c.y; y++) { line((int) xbegin, y, (int) xend, y); xbegin += dbc; xend += dac; } }
Exercise 2: Resizing a Bitmap
/* * simple case of trapezium texture mapping: mapping onto rectangle * (i.e. resizing a bitmap) using DDA. Resized rectangle is assumed * to be represented by: * (x0, y0)+-------+(x1,y0) * | | * | | * (x0, y1)+-------+(x1,y1) */ void resizeBmp(int x0, int y0, int x1, int y1, PImage bmp) { int newBmpWidth = x1 - x0; int newBmpHeight = y1 - y0; double dW = 1.0 * bmp.width / newBmpWidth; double dH = 1.0 * bmp.height / newBmpHeight; double u = 0; for (int i = x0; i < x1; i++) { double v = 0; for (int j = y0; j < y1; j++) { stroke(bmp.get((int) u, (int) v)); point(i, j); v += dH; } u += dW; } }
Exercise 3: Wolfenstein 3D Texture mapping
/* * Assumes trapezium is specified by: * (left, a)+---____ * | ---__+(right, b) * | | * | | * | ___--+(right, c) * (left, d)+___---- */ void drawTrapTexture(int left, int right, int a, int b, int c, int d, PImage bmp) { double dAB = 1.0 * (b - a) / (right - left); double dCD = 1.0 * (c - d) / (right - left); double dX = 1.0 * bmp.width / (right - left); double ytop = a; double ybot = d; double x = 0; double y; for (int i = left; i < right; i++) { double dY = 1.0 * bmp.height / (ybot - ytop); y = 0; for (int j = (int) ytop; j < (int) ybot; j++) { stroke(bmp.get((int)x,(int)y)); point(i,j); y += dY; } ytop += dAB; ybot += dCD; x += dX; } }
Exercise 4: Quake Texture mapping
Look at the source code for the definitions of the auxiliary functions.
void drawQuakeTexture(Point p[], Point tmap[], PImage bmp) { int p1, p2, p3, p4; p1 = p3 = firstPoint(p); p2=nextLeft(p,p1); p4=nextRight(p,p3); do { println("drawing from "+p[p1].x+p[p1].y+" "+p[p2].x+p[p2].y+" "+ p[p3].x+p[p3].y+" "+p[p4].x+p[p4].y); drawLineTexture(p[p1], p[p2], p[p3], p[p4], tmap[p1], tmap[p2], tmap[p3], tmap[p4], bmp); if (p[p2].y < p[p4].y) { p1=p2; p2=nextLeft(p,p1); } else { p3=p4; p4=nextRight(p,p3); } } while (p1!=p3); }
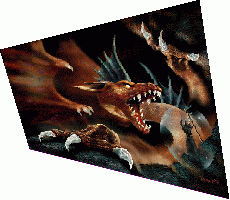